Building an AI-Powered Cobra Wings Exercise Counter App with Pose Estimation Using QuickPose iOS SDK
Are you exploring how to develop an AI-powered Cobra Wings exercise counter for your fitness app? The Cobra Wings exercise is a dynamic movement designed to target the upper back muscles, including the trapezius, rhomboids, and posterior deltoids, while also engaging the core and shoulders. This exercise is effective for improving posture, enhancing shoulder mobility, and strengthening the upper back.
In this guide, you’ll learn how to build a Cobra Wings exercise counter app using pose estimation with the QuickPose iOS SDK. Whether you’re creating a new fitness app or adding advanced features to an existing platform, this tutorial will equip you with the tools to deliver a cutting-edge Cobra Wings counter powered by AI and pose estimation technology.
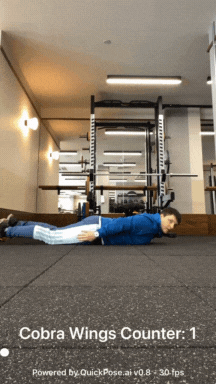
Steps to integrate an AI Cobra Wings Counter into your app:
Register an SDK Key with QuickPose
Get your free SDK key on https://dev.quickpose.ai, usage limits may apply. SDK Keys are linked to your bundle ID, please check Key before distributing to the App Store.
This is a quick look to integrate the cobra wings counter using the QuickPose iOS SDK. You can see the full documentation here: QuickPose iOS SDK Cobra Wings Counter installation.
Activate Cobra Wings Feature
feature = .fitness(.cobraWings)
feature = .fitness(.cobraWings, style: customOrConditionalStyle)
Form Feedback
We recommend using the feature feedback to guide the user if an error occurs.
quickPose.start(features: [.fitness(.cobraWings)], onFrame: { status, image, features, feedback, landmarks in
switch status {
case .success:
overlayImage = image
if let result = features.values.first {
feedbackText = "Cobra Wings: \(Int(result.value * 100))%"
} else if let feedback = feedback.values.first, feedback.isRequired {
feedbackText = feedback.displayString
} else {
feedbackText = nil
}
case .noPersonFound:
feedbackText = "Stand in view";
case .sdkValidationError:
feedbackText = "Be back soon";
}
})
Conditional Styling
To give user feedback consider using conditional styling so that when the user’s measurement goes above a threshold, here 0.8, a green highlight is shown.
let greenHighlightStyle = QuickPose.Style(conditionalColors: [QuickPose.Style.ConditionalColor(min: 0.8, max: nil, color: UIColor.green)])
quickPose.start(features: [.fitness(.cobraWings, style: customOrConditionalStyle)],
onFrame: { status, image, features, feedback, landmarks in ...
})
How to Count Cobra Wing Reps
Real-time Cobra Wing counting with AI
To count the Cobra Wings declare a configurable threshold counter, which can be used to turn lots of our features into counts.
@State private var counter = QuickPoseThresholdCounter()
Then pass QuickPose’s Cobra Wing result to the counter, and display in the feedback text declared above.
quickPose.start(features: [.fitness(.cobraWings)], onFrame: { status, image, features, feedback, landmarks in
switch status {
case .success:
overlayImage = image
if let result = features.values.first {
let counterState = counter.count(result.value)
feedbackText = "\(counterState.count) Cobra Wings"
} else {
feedbackText = nil
}
case .noPersonFound:
feedbackText = "Stand in view";
case .sdkValidationError:
feedbackText = "Be back soon";
}
})
Cobra Wings Timer
To time the Cobra Wings declare a configurable threshold timer, which can be used to turn lots of our features into timers. For Cobra Wings, we suggest modifying the default threshold, taking account of expected camera positioning and tilt.
@State private var timer = QuickPoseThresholdTimer(threshold: 0.2)
Then pass the result’s raw value to the timer, and display in the feedback text declared above.
quickPose.start(features: [.fitness(.cobraWings)], onFrame: { status, image, features, feedback, landmarks in
switch status {
case .success:
overlayImage = image
if let result = features.values.first {
let timerState = timer.time(result.value)
feedbackText = String(format: "%.1f", timerState.time) + "secs"
} else {
feedbackText = nil
}
case .noPersonFound:
feedbackText = "Stand in view";
case .sdkValidationError:
feedbackText = "Be back soon";
}
})