Building an AI-Powered Front Raises Counter App with Pose Estimation Using QuickPose iOS SDK
Are you searching for a way to develop an AI-powered front raises counter for your fitness app? Front raises are a key isolation exercise that primarily targets the anterior deltoids, the front part of the shoulder, while also engaging the upper chest and trapezius muscles. This exercise is essential for building shoulder strength, enhancing shoulder stability, and improving upper body endurance.
In this guide, you’ll learn how to create a front raises counter app using pose estimation with the QuickPose iOS SDK. We’ll walk you through the steps to implement real-time rep counting, ensure accurate form tracking, and provide customised feedback for users. Whether you’re building a new fitness application or upgrading an existing one, this tutorial will help you harness the power of AI and pose estimation technology to deliver a highly effective and user-friendly front raises counter that stands out in the competitive fitness app market.
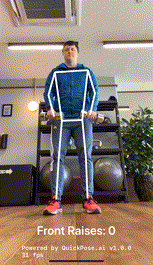
Steps to Count Front Raises in your app with AI:
Register an SDK Key with QuickPose
Get your free SDK key on https://dev.quickpose.ai, usage limits may apply. SDK Keys are linked to your bundle ID, please check Key before distributing to the App Store.
This is a quick look to integrate the front raises counter using the QuickPose iOS SDK. You can see the full documentation here: QuickPose iOS SDK Front Raises Counter installation.
Activate Front Raises Feature
feature = .fitness(.frontRaises)
feature = .fitness(.frontRaises, style: customOrConditionalStyle)
Form Feedback
We recommend using the feature feedback to guide the user if an error occurs.
quickPose.start(features: [.fitness(.frontRaises)], onFrame: { status, image, features, feedback, landmarks in
switch status {
case .success:
overlayImage = image
if let result = features.values.first {
feedbackText = "Front Raises: \(Int(result.value * 100))%"
} else if let feedback = feedback.values.first, feedback.isRequired {
feedbackText = feedback.displayString
} else {
feedbackText = nil
}
case .noPersonFound:
feedbackText = "Stand in view";
case .sdkValidationError:
feedbackText = "Be back soon";
}
})
Conditional Styling
To give user feedback consider using conditional styling so that when the user’s measurement goes above a threshold, here 0.8, a green highlight is shown.
let greenHighlightStyle = QuickPose.Style(conditionalColors: [QuickPose.Style.ConditionalColor(min: 0.8, max: nil, color: UIColor.green)])
quickPose.start(features: [.fitness(.frontRaises, style: customOrConditionalStyle)],
onFrame: { status, image, features, feedback, landmarks in ...
})
How to Count Front Raises
Real-time Front Raises counting with AI
To count the Front Raises declare a configurable threshold counter, which can be used to turn lots of our features into counts.
@State private var counter = QuickPoseThresholdCounter()
Then pass QuickPose’s Front Raise result to the counter, and display in the feedback text declared above.
quickPose.start(features: [.fitness(.frontRaises)], onFrame: { status, image, features, feedback, landmarks in
switch status {
case .success:
overlayImage = image
if let result = features.values.first {
let counterState = counter.count(result.value)
feedbackText = "\(counterState.count) Front Raises"
} else {
feedbackText = nil
}
case .noPersonFound:
feedbackText = "Stand in view";
case .sdkValidationError:
feedbackText = "Be back soon";
}
})
Front Raises Timer
To time the Front Raises declare a configurable threshold timer, which can be used to turn lots of our features into timers. For Front Raises, we suggest modifying the default threshold, taking account of expected camera positioning and tilt.
@State private var timer = QuickPoseThresholdTimer(threshold: 0.2)
Then pass the result’s raw value to the timer, and display in the feedback text declared above.
quickPose.start(features: [.fitness(.frontRaises)], onFrame: { status, image, features, feedback, landmarks in
switch status {
case .success:
overlayImage = image
if let result = features.values.first {
let timerState = timer.time(result.value)
feedbackText = String(format: "%.1f", timerState.time) + "secs"
} else {
feedbackText = nil
}
case .noPersonFound:
feedbackText = "Stand in view";
case .sdkValidationError:
feedbackText = "Be back soon";
}
})